What is Multi-Channel Protocol (MCP)? A Comprehensive Guide for Developers
As AI capabilities expand beyond single-task execution into more complex, orchestrated processes, the need for standardized communication between components has become critical.
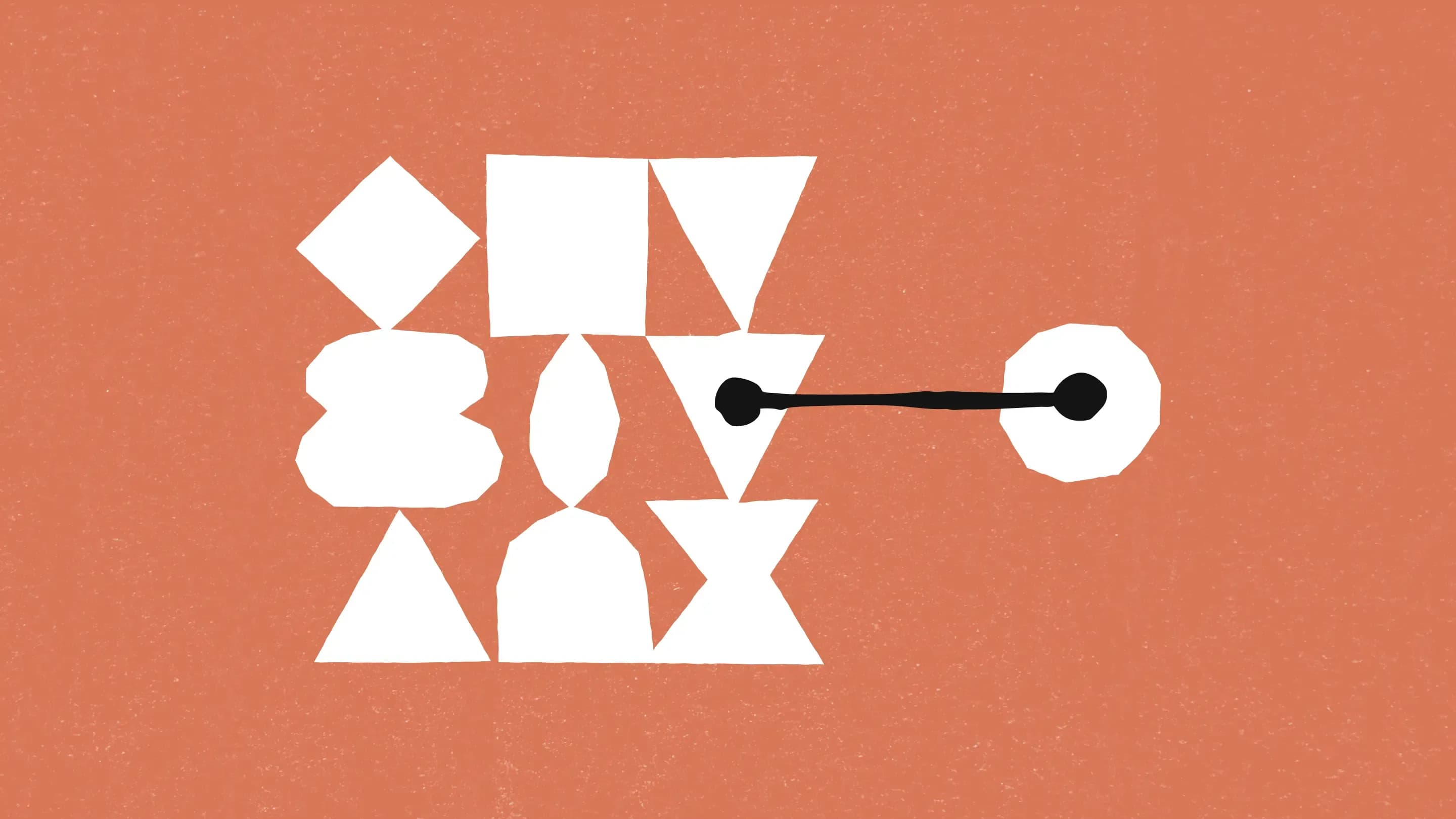
MCP emerged as a response to the limitations of traditional API-based communication when coordinating multiple AI agents, tools, and services. While relatively new in the AI ecosystem, it has quickly gained traction for its ability to solve many challenges in building complex AI workflows.
What is Multi-Channel Protocol (MCP)?
MCP is a communication protocol designed specifically for AI agent orchestration and tool usage. At its core, MCP standardizes how AI components—whether they are language models, specialized tools, or entire agent systems—exchange information, delegate tasks, and coordinate workflows across multiple channels or modalities.
Key Characteristics of MCP
- Multi-Channel Communication: Supports various data types and modalities (text, images, audio, structured data)
- Asynchronous Operations: Enables non-blocking communication between components
- Standardized Interface: Provides consistent patterns for tool registration and function calling
- Dynamic Discovery: Allows agents to discover available tools and capabilities at runtime
- Stateful Conversations: Maintains context across multiple interactions
- Security Controls: Implements authentication and permission models for secure communication
The Architecture of MCP Systems
A typical MCP implementation consists of several core components:
1. MCP Server
The central coordination point that:
- Routes messages between components
- Manages tool registrations
- Handles authentication and permissions
- Maintains session state and context
2. Channels
Specialized pathways for different types of data:
- Text channels: For standard text messages and commands
- Function channels: For structured function calls and responses
- Binary channels: For images, audio, and other binary data
- Event channels: For system notifications and state changes
3. Tool Registry
A directory of available tools where:
- Functions register their capabilities
- Schemas define input and output formats
- Documentation is provided for discovery
- Versioning information is maintained
4. Client SDK
Software development kits that:
- Simplify integration with the MCP ecosystem
- Handle serialization and deserialization
- Manage authentication flows
- Provide type-safe interfaces for function calls
How MCP Works: The Communication Flow
Understanding the typical MCP communication flow helps developers implement compatible systems:
- Tool Registration: Tools register their capabilities (function signatures, schemas) with the MCP server
- Session Initialization: Clients establish authenticated connections to the server
- Tool Discovery: Clients query available tools and their capabilities
- Function Calling: Clients make structured requests to specific tools
- Response Handling: Tools process requests and return formatted responses
- State Management: The server maintains context across multiple interactions
This standardized flow ensures that all components—whether they're custom-built tools, third-party services, or AI models—can interact predictably within the ecosystem.
Implementing MCP in Your AI Flow System
Server-Side Implementation
// Example MCP server setup with Node.js
const express = require('express');
const { MCPServer } = require('mcp-server');
const app = express();
const mcpServer = new MCPServer({
authProvider: new JWTAuthProvider(process.env.SECRET_KEY),
toolRegistry: new ToolRegistry()
});
// Register built-in tools
mcpServer.registerTool('calculator', new CalculatorTool());
mcpServer.registerTool('weatherService', new WeatherServiceTool());
// Mount MCP middleware
app.use('/mcp', mcpServer.middleware());
app.listen(3000, () => {
console.log('MCP Server running on port 3000');
});
Tool Implementation
// Example tool implementation
class CalculatorTool extends BaseTool {
constructor() {
super({
name: 'calculator',
description: 'Performs mathematical calculations',
version: '1.0.0'
});
this.registerFunction({
name: 'add',
description: 'Adds two numbers',
parameters: {
type: 'object',
properties: {
a: { type: 'number' },
b: { type: 'number' }
},
required: ['a', 'b']
},
returns: { type: 'number' }
});
}
async add({ a, b }) {
return a + b;
}
}
Client-Side Integration
// Example client usage
const { MCPClient } = require('mcp-client');
const client = new MCPClient({
serverUrl: 'https://mcp.example.com/mcp',
authToken: 'user-jwt-token'
});
async function performCalculation() {
// Get available tools
const tools = await client.discoverTools();
// Call a function on a tool
const result = await client.callFunction('calculator', 'add', { a: 5, b: 3 });
console.log(`The result is: ${result}`); // Output: The result is: 8
}
Best Practices for MCP Implementation
1. Security First
- Implement strong authentication using JWT or OAuth 2.0
- Define granular permissions for tool access
- Validate all inputs before processing
- Encrypt sensitive data in transit and at rest
// Example of secure tool access
mcpServer.registerTool('sensitiveDataTool', new SensitiveDataTool(), {
requiredScopes: ['data.read', 'data.write'],
rateLimit: { maxRequests: 100, windowMs: 60000 }
});
2. Error Handling
- Provide clear error codes and messages
- Implement proper error status propagation
- Design retry mechanisms for transient failures
- Log detailed error contexts for debugging
try {
const result = await client.callFunction('weatherService', 'getForcast', { city: 'London' });
} catch (error) {
if (error.code === 'FUNCTION_NOT_FOUND') {
// Handle misspelled function name
console.log('Did you mean "getForecast"?');
} else if (error.code === 'VALIDATION_ERROR') {
// Handle schema validation errors
console.log(`Invalid parameters: ${error.details}`);
}
}
3. Versioning
- Implement semantic versioning for tools and functions
- Support backward compatibility
- Provide deprecation notices before removing features
- Consider API versioning at the protocol level
4. Performance Optimization
- Implement connection pooling
- Use binary protocols for large data transfers
- Consider compression for network efficiency
- Optimize serialization/deserialization processes
Real-World Applications of MCP
MCP enables sophisticated AI workflows that were previously difficult to implement:
Autonomous Agent Systems
MCP allows autonomous agents to:
- Delegate specialized tasks to appropriate tools
- Coordinate with other agents
- Maintain conversation context across multiple exchanges
- Dynamically discover new capabilities
Complex AI Flows
Developers can build workflows that:
- Combine multiple AI models with different specializations
- Process and transform data across different modalities
- Handle conditional branching based on intermediate results
- Maintain state across long-running processes
Enterprise Integration
Organizations implement MCP to:
- Connect legacy systems with modern AI capabilities
- Standardize AI service interfaces across departments
- Manage access control to sensitive AI functions
- Monitor and audit AI usage across the organization
Start Building AI Workflows Today
Launch for free, collaborate with your team, and scale confidently with enterprise-grade tools.